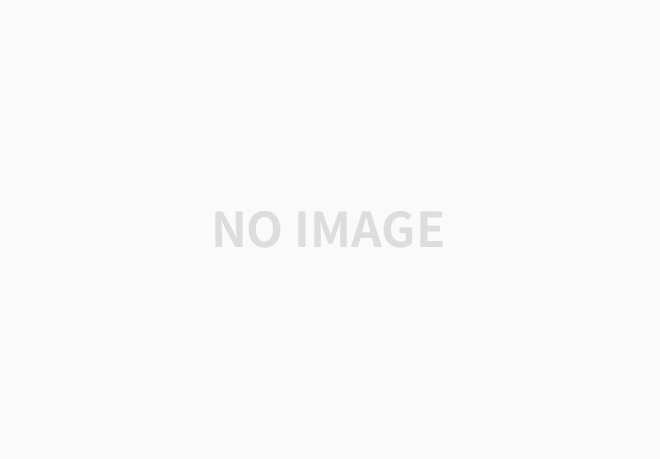
숫자 문자열를 정수형으로 변환하기 위하여 StrToInt(), StrToInt64() 함수를 주로 이용합니다.
숫자 문자열을 다루는 함수들의 원형은 다음과 같습니다.
{ StrToInt converts the given string to an integer value. If the string
doesn't contain a valid value, an EConvertError exception is raised. }
function StrToInt(const S: string): Integer;
function StrToIntDef(const S: string; Default: Integer): Integer;
function TryStrToInt(const S: string; out Value: Integer): Boolean;
{ Similar to the above functions but for Int64 instead }
function StrToInt64(const S: string): Int64;
function StrToInt64Def(const S: string; const Default: Int64): Int64;
function TryStrToInt64(const S: string; out Value: Int64): Boolean;
StrToInt, StrToInt64 함수는 매개변수로 받은 문자열이 숫자만으로 이루어져 있으면, 정수로 변환하여 반환합니다.
하지만, 문자열내에 숫자가 아닌 문자가 포함되어 있으면, EConvertError 오류가 발생합니다.
이를 방지하기 위해서는 try...except... 구문을 이용해야하는 번거로움이 있습니다.
uses SysUtils;
var
strDecimalValue, strHexaValue, strWrongValue: string;
nDecimalValue, nHexaValue: int64;
begin
strDecimalValue := '1234';
strHexaValue := '$abcd';
strWrongValue := 'abcd';
try
nDecimalValue := StrToInt64(strDecimalValue);
nHexaValue := StrToInt64(strHexaValue);
nDecimalValue := StrToInt64(strWrongValue);
except
on EConvertError do writeln('convert error');
end;
writeln(Format('nDecimalValue = %d, nHexaValue = %x', [nDecimalValue, nHexaValue]));
end;
{ output>>
convert error
nDecimalValue = 1234, nHexaValue = ABCD
}
위 코드에서 숫자 문자열 중에서도 16진 숫자 문자열('$abcd' or '0xabcd')일 경우, 맽 앞에 '$'나 '0x'를 포함하여 StrToInt() 함수를 호출하면 16진 문자열을 정수로 변환할 수 있습니다.
StrToInt 또는 StrToInt64는 EConvertError 오류가 발생할 수 있으므로, 다음과 같이 StrToIntDef, StrToInt64Def 함수를 이용하면, try...except... 구문없이 코드를 작성할 수 있습니다.
물론 예외가 발생하지 않는 만큼 조금 더 고민스러운 부분이 있을 수 있지만, StrToInt 또는 StrToInt64 함수를 이용하다가 오류가 발생하여 경고창이 나타나는 것보다는 나을 것입니다.
uses SysUtils;
var
strDecimalValue, strHexaValue1, strHexaValue2, strWrongValue: string;
nDecimalValue1, nDecimalValue2, nHexaValue1, nHexaValue2: int64;
begin
strDecimalValue := '1234';
strHexaValue1 := '$abcd';
strHexaValue2 := '0xabcd';
strWrongValue := 'abcd';
nDecimalValue1 := StrToInt64Def(strDecimalValue, 0);
nDecimalValue2 := StrToInt64Def(strWrongValue, 0);
nHexaValue1 := StrToInt64Def(strHexaValue1, 0);
nHexaValue2 := StrToInt64Def(strHexaValue2, 0);
writeln(Format('nDecimalValue1 = %d, nDecimalValue2 = %d, nHexaValue1 = %x, nHexaValue2 = %x'
, [nDecimalValue1, nDecimalValue2, nHexaValue1, nHexaValue2]));
end;
{ output>>
nDecimalValue1 = 1234, nDecimalValue2 = 0, nHexaValue1 = ABCD, nHexaValue2 = ABCD
}
참고자료
728x90
반응형
'프로그래밍 > 델파이' 카테고리의 다른 글
CCITT CRC16() 함수 (0) | 2021.09.14 |
---|---|
[pascal] 라디오 버튼 선택 동작 (0) | 2021.09.08 |
Lazarus 크로스 컴파일을 통한 Windows 95용 어플 만들기 실패의 기록 (0) | 2021.08.19 |
[pascal] FindWindow / FindWindowEx (0) | 2021.04.01 |
Delphi XE3 64bit UAC 만들기 (0) | 2016.05.22 |